How to Catch S3 Misconfigurations Early with Automated AI Code Reviews

by Atulpriya Sharma
November 26, 2024
11 min read
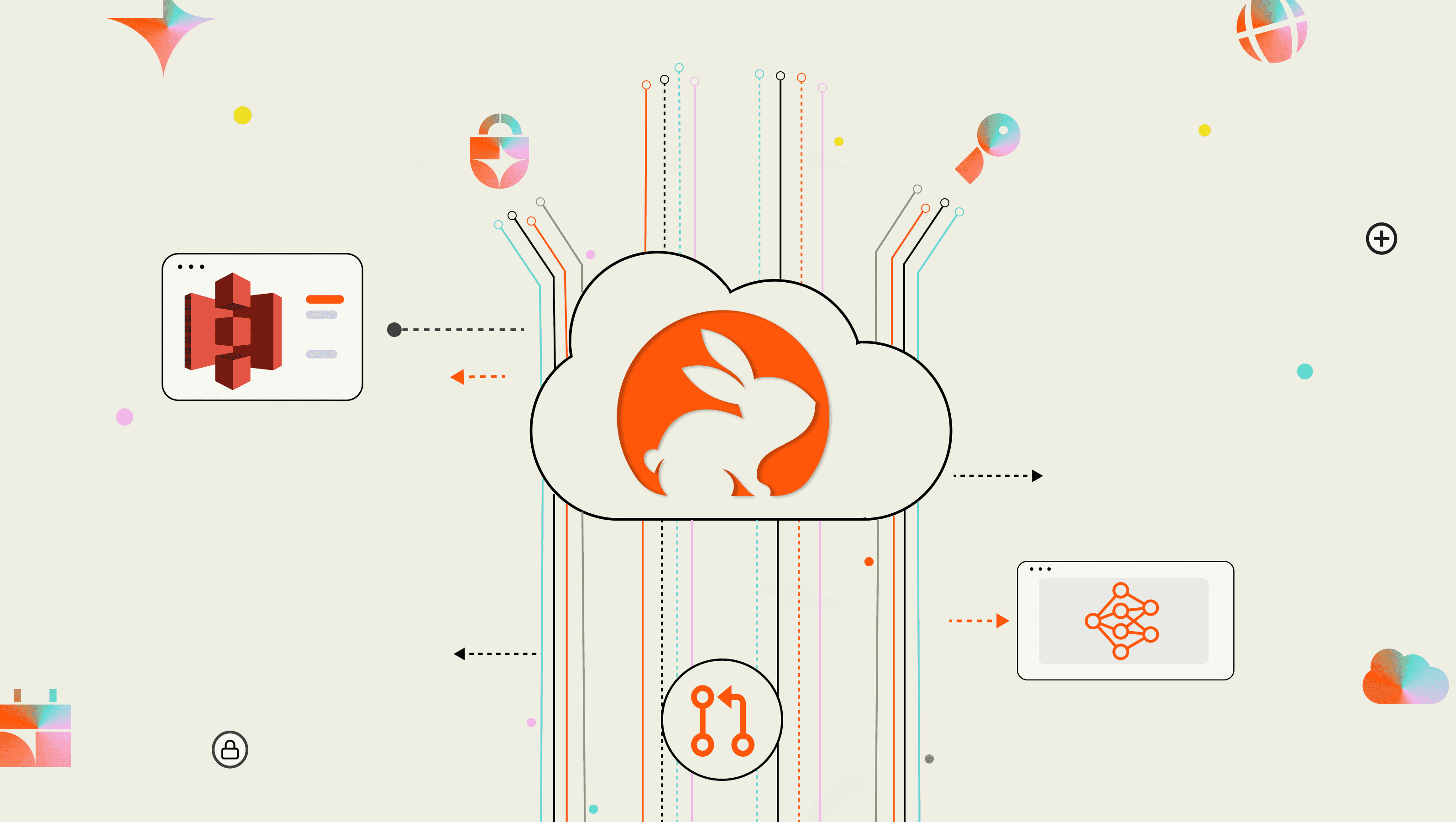
by Atulpriya Sharma
November 26, 2024
11 min read
Most installed AI app on GitHub and GitLab
Free 14-day trial
Most installed AI app on GitHub and GitLab
Free 14-day trial
Still have questions?
Contact us