How to Fix 20 Common PHP Issues With AI

by Aravind Putrevu
November 18, 2024
16 min read
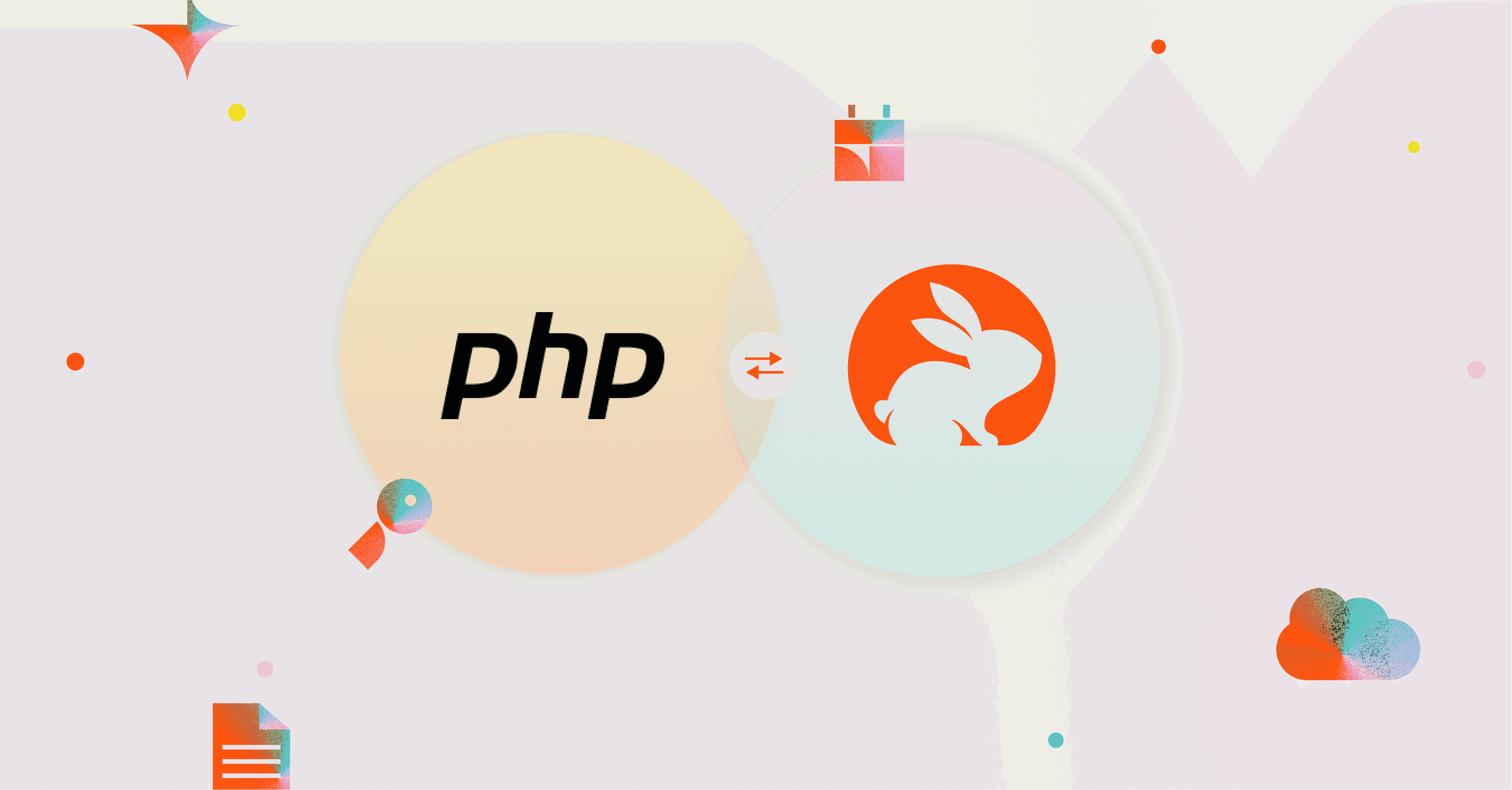
by Aravind Putrevu
November 18, 2024
16 min read
Most installed AI app on GitHub and GitLab
Free 14-day trial
Most installed AI app on GitHub and GitLab
Free 14-day trial
Still have questions?
Contact us