How to Identify and Fix Code Smells in TypeScript using CodeRabbit

by Aravind Putrevu
December 19, 2024
9 min read
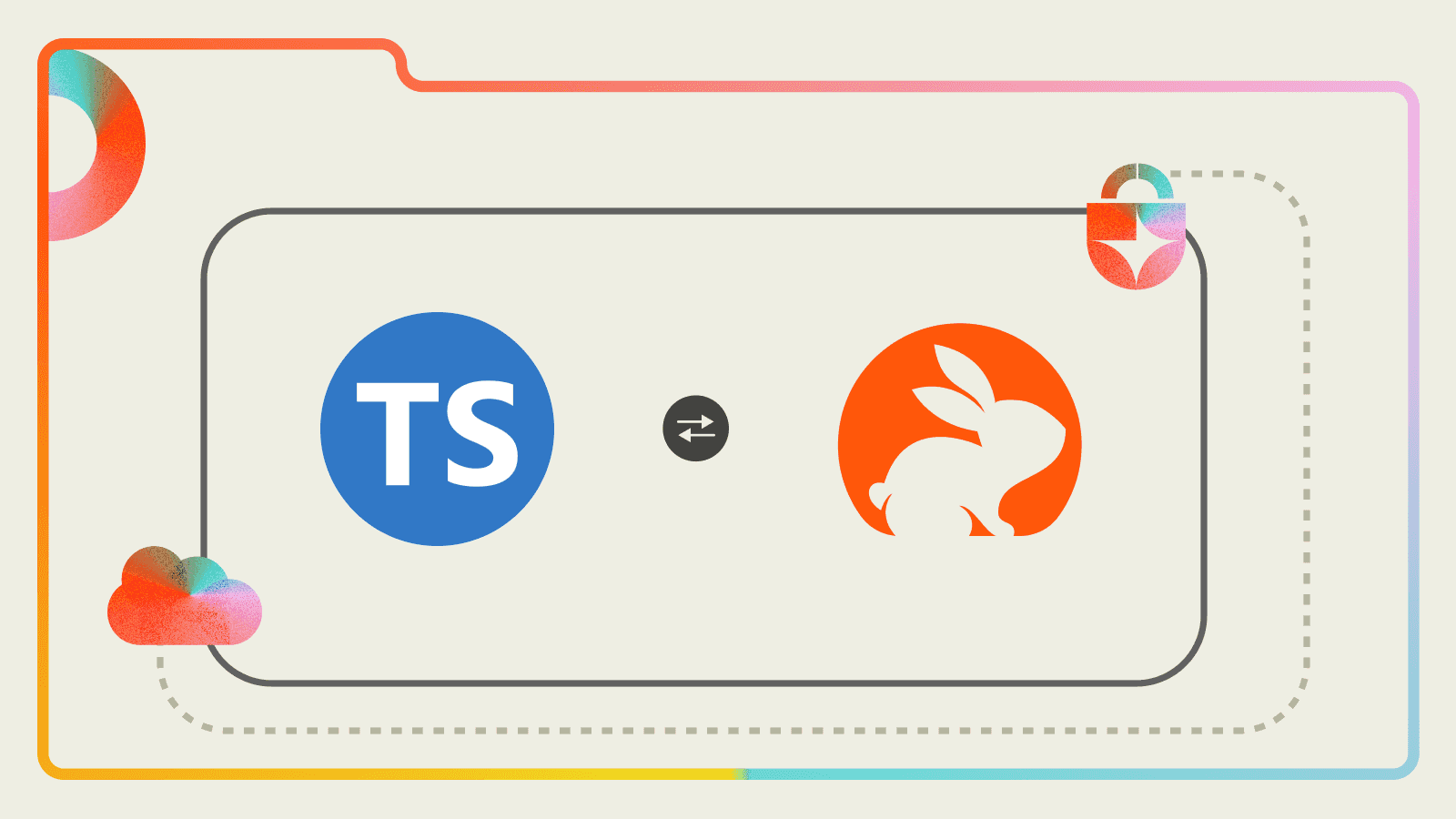
by Aravind Putrevu
December 19, 2024
9 min read
Most installed AI app on GitHub and GitLab
Free 14-day trial
Most installed AI app on GitHub and GitLab
Free 14-day trial
Still have questions?
Contact us