How to Setup Python Code Reviews With CodeRabbit

by Ankur Tyagi
December 04, 2024
9 min read
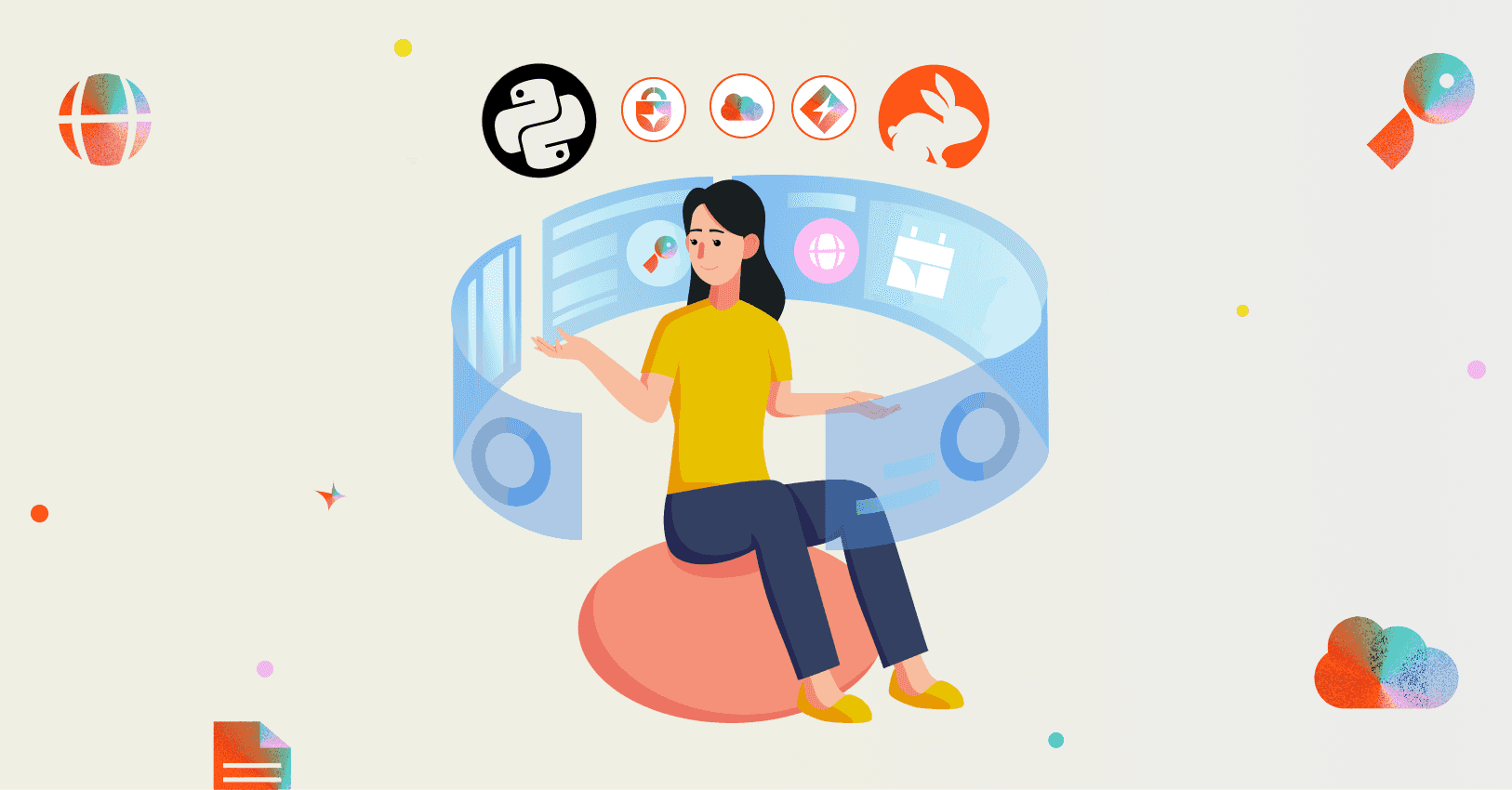
by Ankur Tyagi
December 04, 2024
9 min read
Most installed AI app on GitHub and GitLab
Free 14-day trial
Most installed AI app on GitHub and GitLab
Free 14-day trial
Still have questions?
Contact us